Mastering the 20 Coding Patterns
In today’s competitive job market, coding interviews are becoming harder every day. If you want to stay ahead of the curve, it’s essential to understand how to approach a coding interview. Knowing what interview questions could be asked is only part of the battle; you also need to have the skills to tackle them successfully.
If you’re reading this, you are likely preparing for that all-important coding interview or simply looking to expand your knowledge of algorithms and data structures. Either way, you’re in the right place.
There’s no doubt, a well-prepared candidate is often the successful one. But how can you ensure that you’re fully prepared to take on whatever problem is thrown your way?
This is where pattern recognition comes into play. Recognizing patterns in problem statements can provide valuable insights into how to approach the solution. These programming patterns, like Sliding Window, Two Heaps, etc., serve as a framework for constructing an optimal algorithm. They are the secret tools used by expert programmers to decode complex coding challenges.
In the upcoming sections of this blog, I’ll introduce you to 20 distinct coding interview patterns. You’ll gain insight into the essence of each pattern, its practical usage, and typical problems that can be solved using each pattern. As we demystify each pattern, you’ll soon realize that they’re not as daunting as they initially seem.
By understanding and implementing these patterns, you can develop a robust problem-solving mindset. Whether it’s a startup or big tech companies like Google, Facebook, Amazon, or Microsoft, a candidate’s ability to solve problems efficiently is what interviewers look for. Coding interviews are not about memorizing solutions; they are about problem-solving, critical thinking, and, above all, the correct approach.
Let’s get started.
20 Coding Interview Patterns to the Rescue
Here are the top 20 coding interview patterns discussed in Grokking the Coding Interview:
1. Pattern: Two Pointers
Description: This method uses two pointers to traverse an array or a list from different ends or directions.
Usage: It’s particularly useful for ordered data structures, where we can make intelligent decisions based on the position of the pointers.
Problems: ‘Pair with Target Sum’, ‘Remove Duplicates’, ‘Squaring a Sorted Array’.
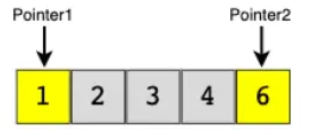
Design Gurus have the most comprehensive list of courses on Coding and System Design interviews for software engineers.
2. Pattern: Island (Matrix Traversal)
Description: It involves traversing a matrix to find ‘islands’ or contiguous groups of elements.
Usage: It’s generally used in grid-based problems, especially when we need to group connected elements together.
Problems: ‘Number of Islands’, ‘Biggest Island’, ‘Flood Fill’.
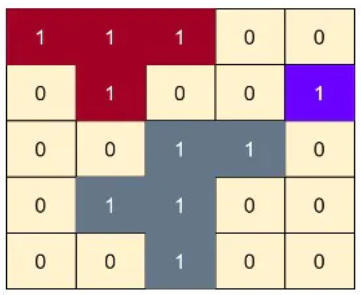
3. Pattern: Fast & Slow Pointers
Description: In this method, two pointers move at different speeds in a data structure.
Usage: It is commonly used to detect cycles in a structure, find middle elements, or to solve other specific problems related to linked lists.
Problems: ‘LinkedList Cycle’, ‘Middle of the LinkedList’, ‘Palindrome LinkedList’.
Grokking the Coding Interview contains a detailed breakdown of these patterns and the LeetCode type questions that can be solved through these patterns. It has a vibrant community of like-minded learners, who share their doubts, discuss ideas, and learn together.
4. Pattern: Sliding Window
Description: This pattern involves creating a ‘window’ into the data structure and then moving that window around to gather specific information.
Usage: Mostly used in array or list-based problems where you need to find a contiguous subset that fulfills certain conditions.
Problems: ‘Maximum Sum Subarray of Size K’, ‘Fruits Into Baskets’, ‘Longest Substring with K Distinct Characters’.
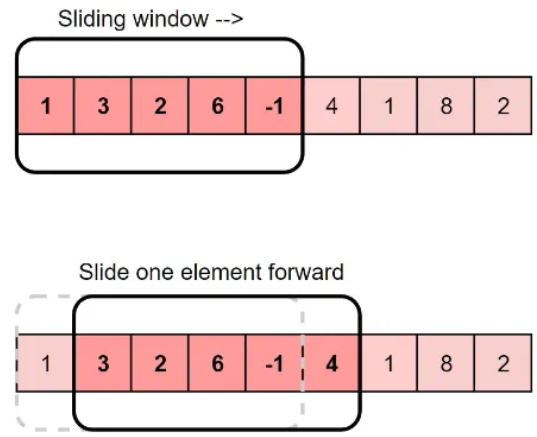
5. Pattern: Merge Intervals
Description: This pattern involves merging overlapping intervals.
Usage: Often used in problems involving time intervals, ranges, or sequences.
Problems: ‘Merge Intervals’, ‘Insert Interval’, ‘Intervals Intersection’.
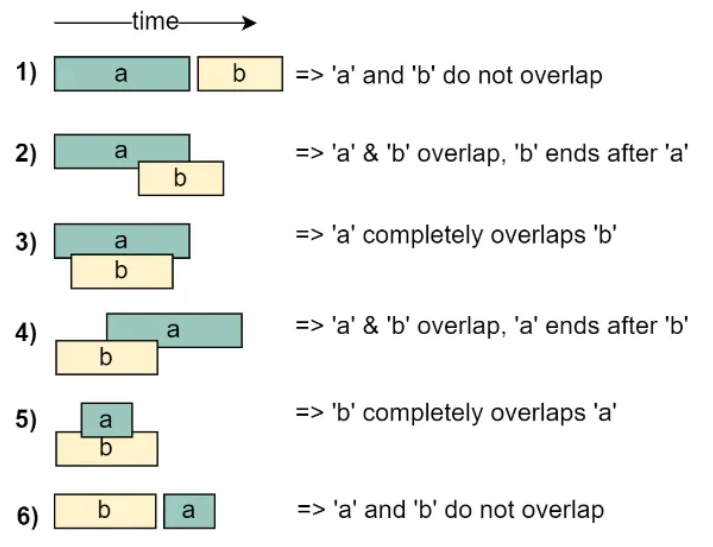
6. Pattern: Cyclic Sort
Description: This pattern involves sorting an array containing numbers in a given range.
Usage: It’s useful in situations where the data involves a finite range of natural numbers.
Problems: ‘Find the Missing Number’, ‘Find all Duplicates’, ‘Duplicates In Array’.
7. Pattern: In-place Reversal of a Linked List
Description: This pattern involves reversing elements of a linked list in-place.
Usage: It’s generally used when reversing a sequence without using extra space i.e., with a constant space complexity.
Problems: ‘Reverse a LinkedList’, ‘Reverse a Sub-list’, ‘Reverse Every K-element Sub-list’.
8. Pattern: Tree Breadth First Search
Description: This pattern involves level-by-level traversal of a tree.
Usage: It’s used when we need to traverse a tree or graph in a level-by-level (breadth-first) manner.
Problems: ‘Binary Tree Level Order Traversal’, ‘Reverse Level Order Traversal’, ‘Zigzag Traversal’.
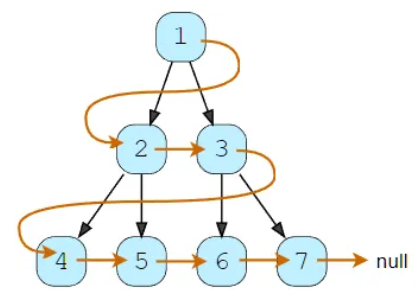
9. Pattern: Tree Depth First Search
Description: This pattern involves traversing a tree or graph depth-wise before visiting siblings or neighbors.
Usage: It’s used when you need to search deeper into a tree/graph first before going across.
Problems: ‘Binary Tree Path Sum’, ‘All Paths for a Sum’, ‘Count Paths for a Sum’.
10. Pattern: Two Heaps
Description: This pattern involves using two heaps to divide a set of numbers into two parts.
Usage: It’s useful when you need to find median numbers in a sequence, or other similar problems.
Problems: ‘Find the Median of a Number Stream’, ‘Sliding Window Median’, ‘Maximize Capital’.
11. Pattern: Subsets
Description: This pattern involves generating all subsets of a set.
Usage: It’s helpful for solving problems that require exploring all subsets of a given set.
Problems: ‘Subsets’, ‘Subsets With Duplicates’, ‘String Permutations’.
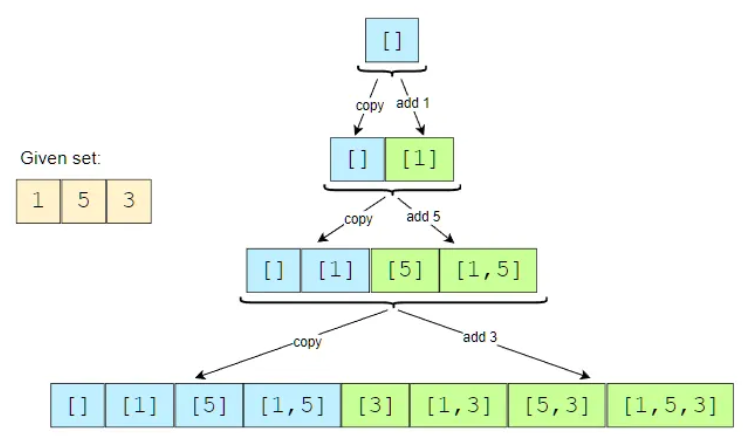
12. Pattern: Modified Binary Search
Description: This is a tweaked version of the binary search algorithm.
Usage: It’s used when a simple binary search isn’t sufficient, like finding a number in a bitonic array.
Problems: ‘Order-agnostic Binary Search’, ‘Ceiling of a Number’, ‘Next Letter’.
13. Pattern: Top ‘K’ Elements
Description: This pattern is used to find the top ‘k’ elements among a certain category.
Usage: It’s commonly used in problems involving sorting, searching, and in heap data structures.
Problems: ‘Top K Frequent Numbers’, ‘Kth Largest Number in a Stream’, ‘Top K Frequent Elements’.
14. Pattern: Bitwise XOR
Description: Bitwise XOR pattern is used to solve various array-based problems.
Usage: It’s used when we need to manipulate and compare bits directly.
Problems: ‘Single Number’, ‘Two Single Numbers’, ‘Complement of Base 10 Number’.
15. Pattern: Backtracking
Description: This pattern involves exploring all possible solutions and then backtracking to correct the course whenever you’re on the wrong path.
Usage: It’s typically used for solving complex combinatorial problems, puzzles, and games.
Problems: ‘Sudoku Solver’, ‘Factor Combinations’, ‘Generate Parentheses’.
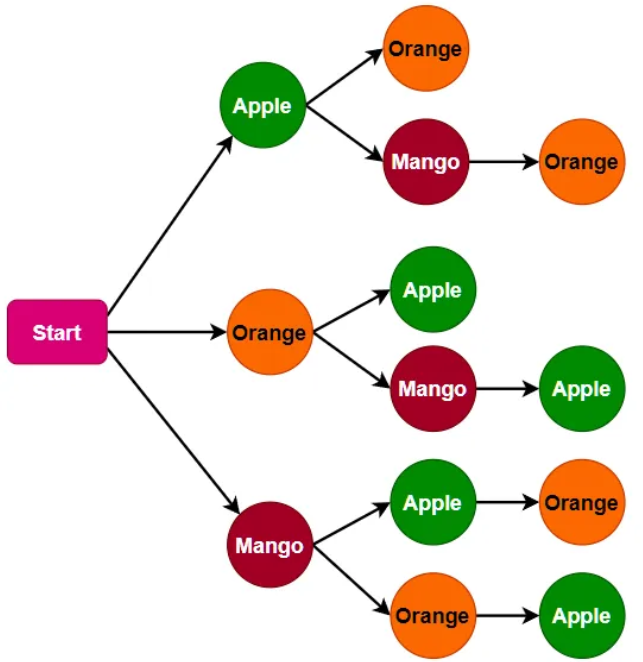
16. Pattern: 0/1 Knapsack (Dynamic Programming)
Description: This pattern deals with problems where items have different values and weights, and we need to determine the maximum value we can carry.
Usage: It’s typically used in optimization problems, especially those involving physical constraints.
Problems: ‘0/1 Knapsack’, ‘Equal Subset Sum Partition’, ‘Subset Sum’.
Check Grokking Dynamic Programming Patterns for Coding Interviews for dynamic programming patterns.
17. Pattern: Topological Sort (Graph)
Description: This pattern involves sorting nodes in a directed graph in a specific order where the preceding node comes before the following node.
Usage: It’s used for scheduling problems and in scenarios where order needs to be imposed on how you process nodes.
Problems: ‘Task Scheduling Order’, ‘All Tasks Scheduling Orders’, ‘Alien Dictionary’.
18. Pattern: K-way Merge
Description: This pattern involves merging ‘k’ sorted lists.
Usage: It’s typically used in problems involving lists, where merging is required.
Problems: ‘Merge K Sorted Lists’, ‘Kth Smallest Number in M Sorted Lists’, ‘Smallest Number Range’.
19. Pattern: Monotonic Stack
Description: This pattern involves using a stack to maintain a monotonic (either entirely non-increasing or non-decreasing) order of elements.
Usage: It’s often used for solving problems where you need to find the next greater or smaller elements.
Problems: ‘Next Greater Element’, ‘Next Smaller Element’, ‘Largest Rectangle in Histogram’.
20. Pattern: Multi-threaded
Description: This pattern involves designing algorithms that can execute multiple threads in parallel.
Usage: It’s used in situations where a task can be divided into independent sub-tasks that can execute concurrently.
Problems: ‘Invert Binary Tree’, ‘Binary Search Tree Iterator’, ‘Same Tree’.
Conclusion
Through this journey, you will discover that these patterns aren’t standalone. They often interconnect and blend into each other, mirroring the complex but exciting challenges in real-world programming. More importantly, you’ll start to see that these patterns not only equip you to perform well in your coding interview but also help you become a better problem solver, a crucial skill in the world of programming.
In this dynamic world of technology, where change is the only constant, having a firm grasp of these timeless patterns will always keep you ahead. They will not only help you crack your software engineer interview but also mold you into a competent and versatile programmer.
So, continue practicing, continue exploring, and continue growing. Don’t be disheartened by the problems that stump you; instead, see them as opportunities to learn and improve. Remember, in coding and life, the journey is just as important as the destination.
I wish you all the very best in your coding adventures. Keep decoding, keep creating, and most importantly, keep enjoying the process! Happy coding!
To learn more about these patterns and the type of questions that can be solved using them, take a look at Grokking the Coding Interview and Grokking Dynamic Programming for Coding Interviews.
Keep learning more about system design and coding interviews:
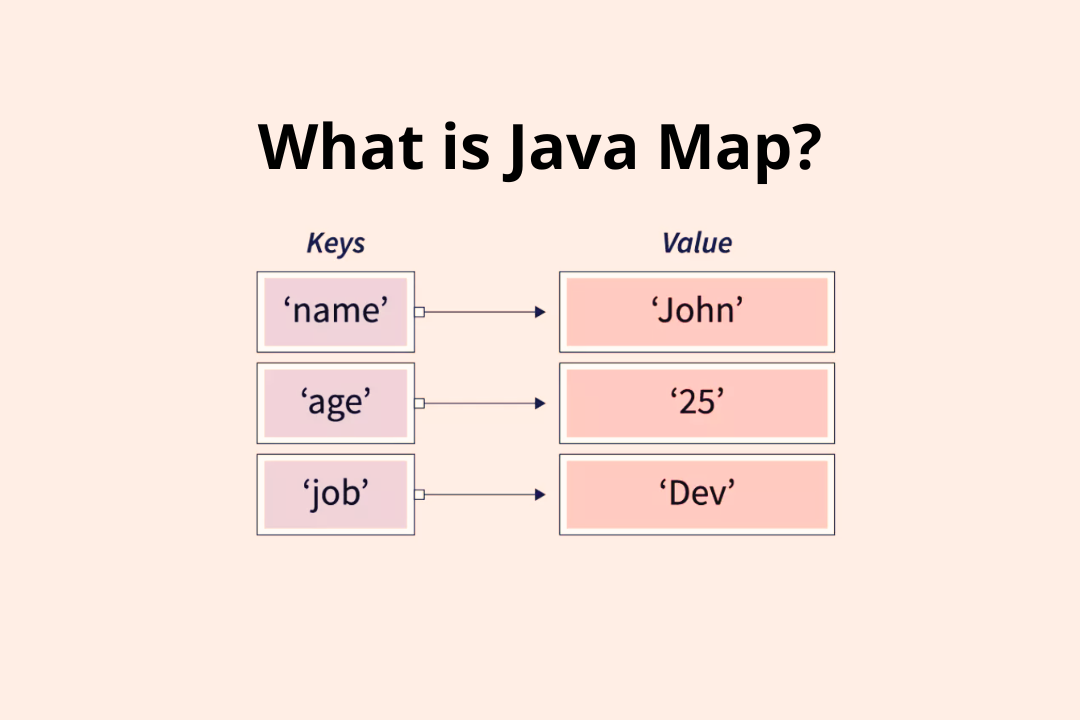
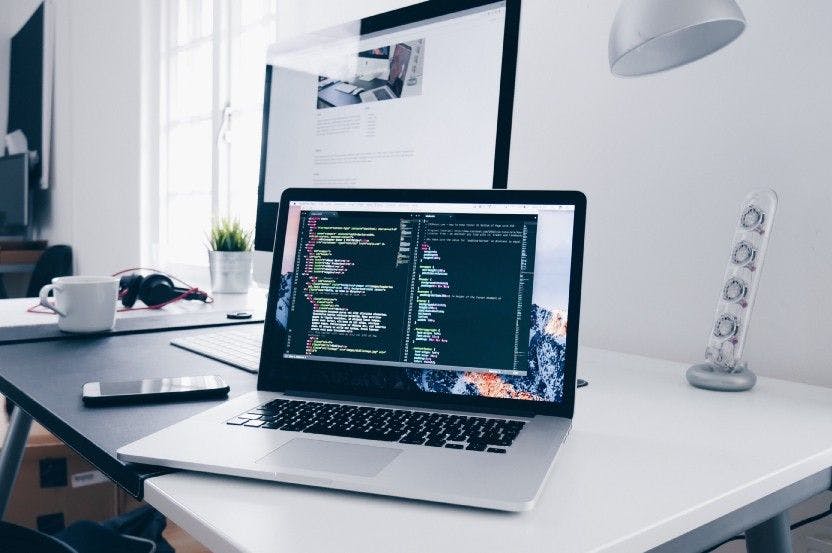