Mastering the UMPIRE Interview Strategy in Coding: A Step-by-Step Guide
Introduction
To get a job as a software developer in a tech company, you need to complete the coding interview. In the fiercely competitive world of tech job interviews, it's crucial to have a well-defined strategy to practice coding interview questions and ace them.
One such strategy that has gained popularity in recent years is the UMPIRE strategy. The UMPIRE method is an acronym that stands for Understand, Match, Plan, Implement, Review, and Expand.
In this blog, we'll explore the UMPIRE interview strategy in coding, breaking down each step with real-time examples to help you navigate your way to success.
What is the UMPIRE strategy?
The UMPIRE strategy helps students and professional developers to solve the coding interview questions. Let's explore the meaning of each letter in UMPIRE.
- U means Understand: When the interviewer asks you the question, you should understand the problem correctly. You may ask the interviewer to give test cases to understand the problem better.
- M means Match: You should match the given problem's pattern with the existing problem patterns before starting to solve the problem. Example problem patterns are two-pointer, Dynamic programming, etc.
- P means Plan: Define the solution approach and try to prepare the pseudo-code.
- I means Implement: Implement the proper code.
- R means Review: After writing the code, review the code and check for errors. Also, check the code's output for edge cases.
- E means Evaluate: The last step is to evaluate the performance of the algorithm using the time and space complexity. Also, you may look at further optimization of the code.
Also, look at Grokking coding interview: Pattern for coding questions.
UMPIRE Example
Now, let's understand how to use the UMPIRE strategy while solving real-time coding questions.
Problem: Pair with the target sum
Statement: You have given an array arr
containing multiple numbers and a numeric value targetSum
. It is also given that an array arr
is sorted in ascending order. The given task is to the pair of two array elements such that their sum is equal to the targetSum
. You need to return the array of size 2 containing the indices of elements forming the valid pair.
Now, let's follow the UMPIRE strategy to solve the given question.
Understand
The best way to understand the problem is by clarifying the problem statement and understanding the output of the test cases.
The problem statement of the given coding question is self-explanatory. So, let's explore the test cases.
Input: arr = [1, 2, 3, 4, 5, 6], targetSum = 6 output: [1, 3]
Also, you should look into the edge test cases, as shown below.
Input: arr = 4], targetSum = 4 output: []
Here, we have considered the empty array as an edge test case. In this case, it should return the empty array. Also, you should clarify what answer is expected when an array contains a single element.
Furthermore, you could ask questions given below to the interviewer for more clarification.
- What is the time and space complexity expected from the solution?
- Should I use a specific problem pattern to solve the problem?
- What to do when an array contains multiple valid pairs?
You can take 4 to 5 minutes to understand the question.
Match
The next step is to identify the pattern of the question. In the coding interviews of most tech companies, you will never be asked repeated questions, but they ask questions of similar patterns. Here, the coding question pattern refers to various approaches to solve the problem, like two pointers, fast & slow pointers, sliding windows, merge intervals, cyclic sort, two heaps, breadth-first search, depth-first search, etc.
Furthermore, you are also required to choose the proper data structure to solve the problem. For example, if you need to perform searching, you can use a sorted array or binary tree. You can use the queue to get output in sorted order.
For the given problem, you can consider the matching factors given below.
- Data structure: Array or HashMap
- Pattern: Binary search and Two-pointer
The matching part should not take more than 5 minutes.
Plan
The planning stage is one of the most important as you build the logic to solve the problem in this part.
First, you should think about different approaches to solving the problem. The given problem can be solved using three different approaches as given below.
- Using the binary search algorithm
- Using the two pointers approach
- Using the Hashmap data structure
You may ask the interviewer if they need you to solve the question using a particular approach. Otherwise, you can pick one of the most efficient approaches according to you.
Here, we will use the two-pointer approach to solve the problem as the given array is sorted and write a pseudo code. Basically, you need to develop the algorithm for the problem solution.
Pseudo-code
def search(self, arr, target_sum): # Traverse array until the left pointer is less than the right pointer while(left < right): # When you find a valid pair, return it if arr[left] + arr[right] == target_sum: return [left, right] if target_sum > current_sum: left += 1 # We need a pair with a bigger sum else: right -= 1 # We need a pair with a smaller sum return [-1, -1] # When the valid solution doesn't exist.
Algorithm
Now, let’s understand the pseudo-code using the algorithm given below.
- Initialize the
’left’ pointer with 0 and the
rightpointer with
array_len – 1`. - Use the loop to traverse the array until the value of the
left
pointer is less than theright
pointer. - In the loop, if the sum of the elements at the
left
andright
index is equal totargetSum
, return[left, right]
. - If the sum of elements is less than the
targetSum
, increment theleft
pointer by 1. Otherwise, increment theright
pointer by 1. - In the end, return
[-1, -1]
if valid pair doesn’t exist.
You can write the pseudo-code in the paper. It might not be easy for everyone to get an instant idea about the solution. So, you can think wisely and take up to 10 to 15 minutes to find the proper approach.
Implementation
Sometimes, it is enough to explain the logic of the question and write the pseudo-code due to a shortage of time in the interview, but implementing the whole code can help you stand out from the crowd.
Some interviewers ask you to implement the code in the code editor.
Solution:
class Solution: def search(self, arr, targetSum): left, right = 0, len(arr) - 1 while(left < right): currentSum = arr[left] + arr[right] if currentSum == targetSum: return [left, right] if targetSum > currentSum: left += 1 else: right -= 1 return [-1, -1] def main(): sol = Solution(); print(sol.search([1, 2, 3, 4, 6], 6)) print(sol.search([], 1)) main()
[1, 3] [-1, -1]
The implementation part can take up to 5 to 7 minutes, according to your programming language knowledge and skills.
Review
It is very important to review the code after writing. Generally, most coders skip this part. By reviewing your code, you can check whether your code is suitable to handle all test cases and give correct output.
Let’s run our code by dry-running it with sample test cases.
- The given input array is [1, 2, 3, 4, 5, 6], and
targetSum
is 6. - When we run the code, the initial value of the
left
pointer is 0, and theright
pointer
is 4. - Next, the loop will start iteration as 0 is less than 5.
- In the first iteration,
currentSum
will be 7 (6 +1). - The
currentSum
is greater than 6, so it will decrementright
by 1. Now,right
is 3. - Now, 0 is less than 3, so the loop will continue iteration.
- The value of the
currentSum
is 5 (4 + 1), which is less than thetargetSum
. So, it will incrementleft
by 1. - Next, 1 is less than 3, so the loop will continue iteration.
- In this iteration,
currentSum
is 6, which is equal totargetSum
. So, the code will return[left, right]
.
Handling Edge Cases
Let’s try to dry-run the second input in which the size of the array is 0.
- The initial value of the
left
andright
pointer will be 0. - Here,
left
andright
are the same so that the loop won’t make any iteration. - At the end, it will return
[-1, -1]
.
The code seems perfect and able to handle each test case. If you find any error while reviewing, you can correct the error.
Evaluate
The final step of the UMPIRE strategy is to discuss the efficiency, advantages, disadvantages, and why you have chosen the algorithm with your interviewer.
Also, you can discuss if your code can accept only a specific type of input. For example, here, the code takes a sorted array as input. If you pass an unsorted array as an input, it can give you the wrong output.
Time complexity evaluation
In the solution code, the loop traverses through the array and makes N – 1 iteration in the worst-case scenario. So, the time complexity of the code is O(N).
Space complexity
In the solution code, we don’t use any extra space. So, the space complexity of the code is O(1).
You can also discuss other algorithms and techniques to solve the given problem, like binary search and using a hashtable. For example, the time complexity of using the binary search algorithm is O(NlogN), and if you use the hashtable, it takes O(N) space. So, the two-pointer approach is more efficient than other approaches.
Want to prepare for your next coding interview? Look at Coding Interview RoadMap by Designguru.
Also, look at Grokking Dynamic Programming Patterns for Coding Interviews.
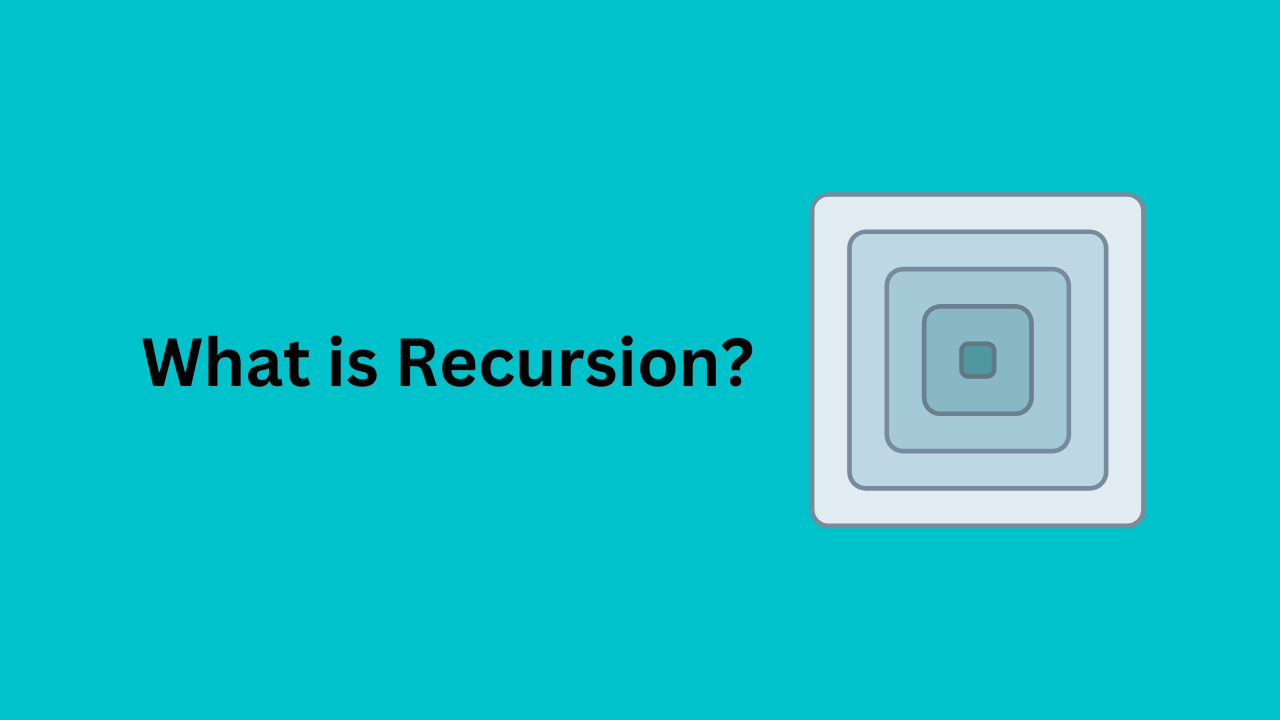